I’ve been working on the Prismatik code to get it ready to go back onto the main branch so I thought I’d post a little update.
One of the Pull Request suggestions was to replace my hacked Adalight protocol with one of the protocols listed here. This would then allow the Prismatik software to control LEDs connected to a WLED device.
Rather than pick a single protocol from the list, we decided it would be best to implement all of them as new devices. So now when you configure a new device, you’re presented with “DRGB”, “DNRGB” and “WARLS” as options.
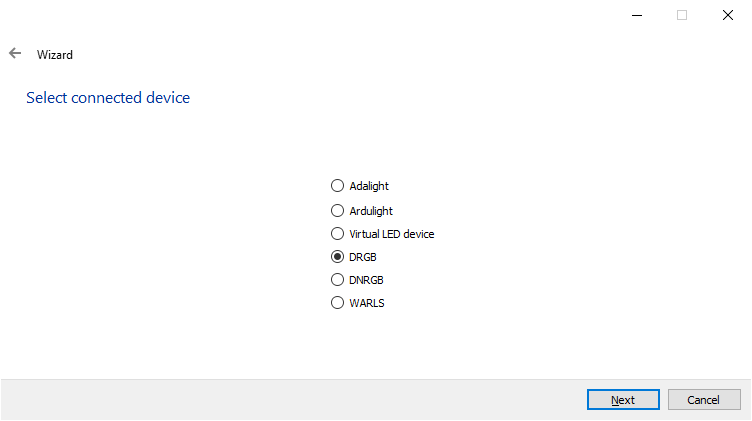
I also added a configuration page that lets you specify what IP address and port you want to send the data to.
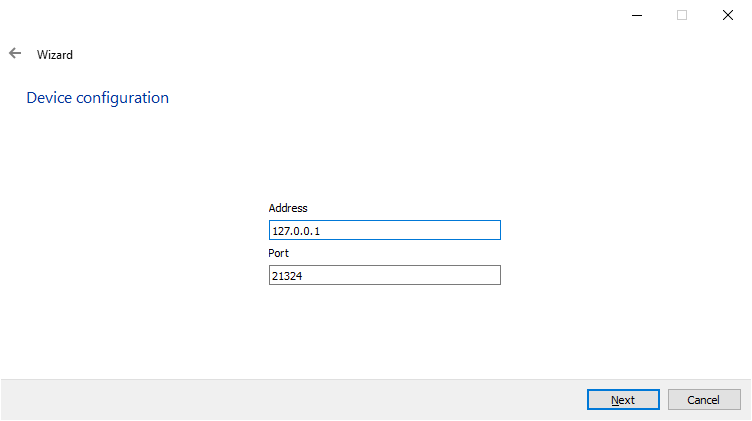
A nice side effect is that the Audio Reactive LED Strip (ARLS) code (that I’ve worked with before) can be tweaked to output in the WARLS format. So I updated my Python script and ran the ARLS software for an audio sync’d Ambilight:
While Prismatik offers an audio sync mode, ARLS has a few more options that make it look a bit cooler. My favourite mode (shown above) has the colours start in the top-middle of the screen and run around the edges.
I’m working on tidying up the last bits of code, but hopefully next time you download Prismatik you’ll have all of the new device options to choose from.
Here’s the latest Python script for the Mote that supports both Prismatik and ARLS.
from socket import *
import atexit
from mote import Mote
leds = 64
pixels = 16
gamma_correction = False
translate_led = True
mote = Mote()
mote.configure_channel(1, pixels, gamma_correction)
mote.configure_channel(2, pixels, gamma_correction)
mote.configure_channel(3, pixels, gamma_correction)
mote.configure_channel(4, pixels, gamma_correction)
mote.clear()
recvSocket = socket(AF_INET, SOCK_DGRAM)
recvSocket.bind(('', 21324))
led_map = [ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15,
16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31,
47, 46, 45, 44, 43, 42, 41, 40, 39, 38, 37, 36, 35, 34, 33, 32,
63, 62, 61, 60, 59, 58, 57, 56, 55, 54, 53, 52, 51, 50, 49, 48]
while True:
message, address = recvSocket.recvfrom(1024)
# WARLS
for i in range(2, len(message), 4):
if translate_led:
led = led_map[message[i]]
else:
led = message[i]
mote.set_pixel(led//16+1, led%16, message[i+1], message[i+2], message[i+3])
mote.show()
@atexit.register
def close():
recvSocket.close()
Code language: Python (python)